-
Overview of ‘C’ Language
-
C Language Processing Systems & Memory Regions
-
Structure of C Program
-
Errors during Compilation & Runtime Errors
Tutors
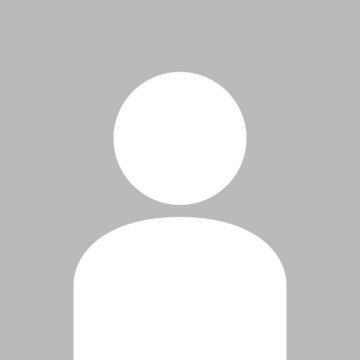
eKalasaala
PROGRAM OVERVIEW
C has proved to be a powerful and flexible language that can be used for a variety of applications, from business programs to engineering. C is a particularly popular language for personal computer programmers because it is relatively small -- it requires less memory than other languages.
The first major program written in C was the UNIX operating system, and for many years C was considered to be inextricably linked with UNIX. Now, however, C is an important language independent of UNIX.
Although it is a high-level language, C is much closer to assembly language than are most other high-level languages. This closeness to the underlying machine language allows C programmers to write very efficient code. The low-level nature of C, however, can make the language difficult to use for some types of applications.
CURRICULUM
Introduction to 'C' Language
Installation Procedure of C Compiler
-
Installation Procedure of C Compiler
Basics of C
-
C Tokens
-
Data Types in C
-
Modifiers & Format Specifiers
-
First C Program using printf & scanf
-
Escape Sequences & Comments in C
Operators
-
Types of Operators and Arithmetic Operators
-
Relational Operator
-
Logical Operator
-
Assignment Operator
-
Increment and Decrement Operator
-
Conditional and Special Operator
-
Bitwise Operator
-
Operators Precedence and Evaluation of Expressions
Selectors
-
Simple if and if..else Statement
-
Nested if and if..else ladder Statement
-
Go-to Statement
-
Switch case Statement
Iterations
-
While Loop
-
Do-while Loop
-
For Loop Lecture-1
-
For Loop Lecture-2
-
Nested Loop Statements Lecture-1
-
Nested Loop Statements Lecture-2
-
Nested Loop Statements Lecture-3
-
Use of Break & Continue Statements in Loops
Arrays
-
Introduction to Arrays
-
Memory Storage of Arrays
-
Types of Arrays and One-Dimensional Arrays
-
Two-Dimensional Arrays
-
Three-Dimensional Arrays
Pointers
-
Introduction to Memories and Pointers
-
Declaration and Initialization of Pointers
-
Using the Pointers
-
Operations on Pointers Lecture -1
-
Operations on Pointers Lecture -2
-
Operations on Pointers Lecture - 3
Functions
-
Introduction to Functions
-
Function Prototype and Defining Function
-
Calling a Function
-
Classification of Functions Lecture - 1
-
Classification of Functions Lecture - 2
-
Classification of Functions Lecture - 3
-
Classification of Functions Lecture - 4
-
Parameter Passing Techniques Lecture - 1
-
Parameter Passing Techniques Lecture - 2
Recursive Functions
-
Recursive Functions Lecture – 1
-
Recursive Functions Lecture – 2
-
Recursive Functions Lecture – 3
Storage Class Specifiers
-
Introduction & Automatic Specifier
-
Register Specifier
-
Static Specifier
-
External Specifier
-
Local and Global Variables
Engineering Mathematics : Basics of Matrices
-
Entering Matrix into 2 - Dimensional Array
-
Identity Matrix
-
Transpose of a Matrix Lecture - 1
-
Transpose of a Matrix Lecture - 2
-
Transpose of a Matrix Lecture - 3
Engineering Mathematics : Matrix Operations
-
Matrix Addition
-
Matrix Subtraction
-
Matrix Multiplication - Code Logic Explanation
-
Matrix Multiplication - Code Implementation
Engineering Mathematics : Determinant and Cofactors of a Matrix
-
Introduction to Determinant
-
Program to Find Determinant
-
Program to find Determinant using Recursion - Code Logic Explanation
-
Program to Find Determinant using Recursion - Code Implementation
-
Cofactors of a Matrix
-
Program on Cofactors of a Matrix
Engineering Mathematics : Creation of User Defined Header Files
-
Creating Determinant User Defined Header File
-
Creating Cofactors User Defined Header File
-
Creating Transpose User Defined Header File
Engineering Mathematics : Inverse of a Matrix
-
Introduction to Inverse of a Matrix
-
Program on Inverse of a Matrix
Engineering Mathematics : Linear Equations
-
Introduction to Linear Equations
-
Linear Equation in Matrix Form - Code Logic Explanation
-
Linear Equation in Matrix Form - Code Implementation
-
Creating Inverse User Defined Header File
-
Solving Linear Equations - Code Logic Explanation
-
Solving Linear Equations - Code Implementation
Electrical & Electronics Application : Mesh Analysis
-
Introduction to Mesh
-
Mesh Current Method
-
Solving Mesh Equations using C
Electrical & Electronics Application : Nodal Analysis
-
Introduction to Node
-
Node Voltage Method
-
Solving Node Equations using C